자바스크립트를 이용해서 간단하게 토스트 팝업을 구현해 보도록 하겠다. 토스트 팝업은 버튼을 클릭하거나 액션을 취하면 알림창 처럼 팝업 화면이 작게 나오는 것이다. 아래 화면을 보면 저런 식으로 나온다.
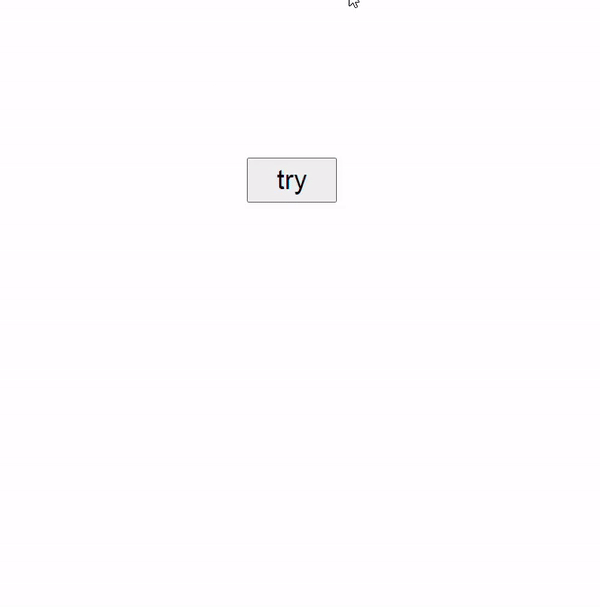
해당 기능을 직접 구현 할 수도 있으나 잘 만들어 놓은 라이브러리가 있다. 그래서 그걸로 시간을 단축해서 사용해 보도록 하겠다.
1. Toastify.js 사용하기
토스트 팝업을 구현하기 위해 Toastify.js라는 라이브러리를 사용할 것 이다.
아래의 사이트에서 어떻게 사용하는지 잘 알 수 있다.
https://github.com/apvarun/toastify-js
GitHub - apvarun/toastify-js: Pure JavaScript library for better notification messages
Pure JavaScript library for better notification messages - apvarun/toastify-js
github.com
1-1. 라이브러리 설정
먼저 index.html 파일을 하나 만들어 준다. 그런 다음 toastify.js를 위한 css와 script를 불러온다.
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>toastify-js-test</title>
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/npm/toastify-js/src/toastify.min.css">
</head>
<body>
<div>
</div>
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/toastify-js"></script>
</body>
</html>
1-2. Toastify 함수 설정
그런 다음 toastify를 실행 할 수 있는 hello라는 함수를 하나 만들어 준다. (나는 index.html에서 바로 사용할 거라 따로 js파일을 안 만들고 그냥 바로 쌩으로 body태그 안에 넣어주겠다.)
<script type="text/javascript">
function hello() { Toastify({
text: "hello toast",
duration: 3000,
gravity: 'bottom',
position: 'center',
style:{
background: "linear-gradient(to right, #00b09b, #96c93d)",
}
}).showToast();};
</script>
간단히 toastify 속성에 대해 설명하자면...
- text - 팝업창에 나올 텍스트
- duration - 팝업 생성 화면 지속시간
- gravity - 어느쪽에서 부터 나올 건지 (top, bottom 이렇게 있다.)
- position - 위치는 어디로 둘 건지 (left, right 이렇게 있다.)
- style - 팝업창 스타일 지정
기본적으로 이런 속성을 사용하며 자세한 내용은 위에 있는 git 사이트의 공식 문서를 참조하면 된다.
1-3. Toastify 사용
이제 함수도 만들었으니 직접 사용해보도록 하겠다. try라는 버튼을 하나 만들어 주고 버튼을 클릭했을 때 토스트 팝업창이 나오도록 해보겠다. 아래는 최종 코드이며 이렇게 하면 맨 처음에 설명한 화면처럼 나온다.
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>toastify-js-test</title>
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/npm/toastify-js/src/toastify.min.css">
</head>
<body>
<div style="display: flex; justify-content: center; align-items: center; height: 100%;">
<button onclick="hello()" style="width: 100px; height: 50px; font-size: 30px;"> try </button>
</div>
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/toastify-js"></script>
<script type="text/javascript">
function hello() { Toastify({
text: "hello toast",
duration: 3000,
gravity: 'bottom',
position: 'center',
style:{
background: "linear-gradient(to right, #00b09b, #96c93d)",
}
}).showToast();};</script>
</body>
</html>
다음에는 react-toastify도 있던데 이걸 한번 사용해서 react에서도 토스트 팝업을 구현해 보도록 하겠다.
자바스크립트를 이용해서 간단하게 토스트 팝업을 구현해 보도록 하겠다. 토스트 팝업은 버튼을 클릭하거나 액션을 취하면 알림창 처럼 팝업 화면이 작게 나오는 것이다. 아래 화면을 보면 저런 식으로 나온다.
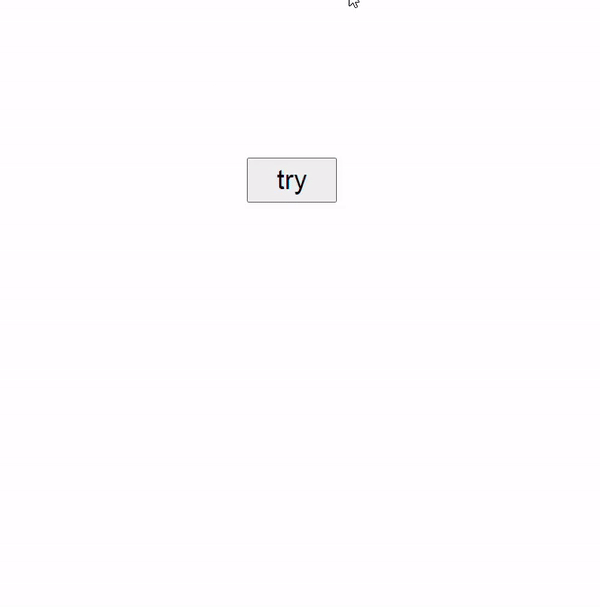
해당 기능을 직접 구현 할 수도 있으나 잘 만들어 놓은 라이브러리가 있다. 그래서 그걸로 시간을 단축해서 사용해 보도록 하겠다.
1. Toastify.js 사용하기
토스트 팝업을 구현하기 위해 Toastify.js라는 라이브러리를 사용할 것 이다.
아래의 사이트에서 어떻게 사용하는지 잘 알 수 있다.
https://github.com/apvarun/toastify-js
GitHub - apvarun/toastify-js: Pure JavaScript library for better notification messages
Pure JavaScript library for better notification messages - apvarun/toastify-js
github.com
1-1. 라이브러리 설정
먼저 index.html 파일을 하나 만들어 준다. 그런 다음 toastify.js를 위한 css와 script를 불러온다.
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>toastify-js-test</title>
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/npm/toastify-js/src/toastify.min.css">
</head>
<body>
<div>
</div>
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/toastify-js"></script>
</body>
</html>
1-2. Toastify 함수 설정
그런 다음 toastify를 실행 할 수 있는 hello라는 함수를 하나 만들어 준다. (나는 index.html에서 바로 사용할 거라 따로 js파일을 안 만들고 그냥 바로 쌩으로 body태그 안에 넣어주겠다.)
<script type="text/javascript">
function hello() { Toastify({
text: "hello toast",
duration: 3000,
gravity: 'bottom',
position: 'center',
style:{
background: "linear-gradient(to right, #00b09b, #96c93d)",
}
}).showToast();};
</script>
간단히 toastify 속성에 대해 설명하자면...
- text - 팝업창에 나올 텍스트
- duration - 팝업 생성 화면 지속시간
- gravity - 어느쪽에서 부터 나올 건지 (top, bottom 이렇게 있다.)
- position - 위치는 어디로 둘 건지 (left, right 이렇게 있다.)
- style - 팝업창 스타일 지정
기본적으로 이런 속성을 사용하며 자세한 내용은 위에 있는 git 사이트의 공식 문서를 참조하면 된다.
1-3. Toastify 사용
이제 함수도 만들었으니 직접 사용해보도록 하겠다. try라는 버튼을 하나 만들어 주고 버튼을 클릭했을 때 토스트 팝업창이 나오도록 해보겠다. 아래는 최종 코드이며 이렇게 하면 맨 처음에 설명한 화면처럼 나온다.
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>toastify-js-test</title>
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/npm/toastify-js/src/toastify.min.css">
</head>
<body>
<div style="display: flex; justify-content: center; align-items: center; height: 100%;">
<button onclick="hello()" style="width: 100px; height: 50px; font-size: 30px;"> try </button>
</div>
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/toastify-js"></script>
<script type="text/javascript">
function hello() { Toastify({
text: "hello toast",
duration: 3000,
gravity: 'bottom',
position: 'center',
style:{
background: "linear-gradient(to right, #00b09b, #96c93d)",
}
}).showToast();};</script>
</body>
</html>
다음에는 react-toastify도 있던데 이걸 한번 사용해서 react에서도 토스트 팝업을 구현해 보도록 하겠다.